App Name : ZYX
Apple ID : 505193110
Bundle Identifier : org.JODI.zyx
Localizations : ( "en" )
Prerendered Icon Flag : true
Original Zip File Name : ZYX.ipa
Bundle Short Version String : 1.2
Bundle Version : 1.2
Minimum OS Requirements : 5.0
Build SDK : 9B176
Build Platform : 9B176
Required Capabilities : armv7
Supported Architectures : armv7
Device Family : iPhone / iPod Touch
Newsstand App : false
Entitlements : ZYX.app/ZYX
get-task-allow: false
keychain-access-groups: ( FQK7H3AF9T.org.JODI.zyx )
application-identifier: FQK7H3AF9T.org.JODI.zyx
#pragma mark - Gyroscope
-(void)updateMotion;{
oldHeading = 0;
offsetG = 0;
newCompassTarget = 0;
if (!locationManager) locationManager=[[CLLocationManager alloc] init];
locationManager.desiredAccuracy = kCLLocationAccuracyBest;
locationManager.delegate=self;
[locationManager startUpdatingHeading];
if (!motionManager) motionManager = [[CMMotionManager alloc] init];
if (!currentQueue) currentQueue = [[NSOperationQueue alloc] init];
motionManager.deviceMotionUpdateInterval = 0.1;
if(motionManager.isDeviceMotionAvailable) {
[motionManager startDeviceMotionUpdatesToQueue:[NSOperationQueue currentQueue]
withHandler: ^(CMDeviceMotion *motion, NSError *error)
{
if(i > 6){
currentRotation = newRotation;
i=0;
}
CMAttitude *currentAttitude = motion.attitude;
float yawValue = currentAttitude.yaw;
float yawDegrees = CC_RADIANS_TO_DEGREES(yawValue);
currentYaw = yawDegrees;
if([CLLocationManager headingAvailable] == YES){
yawDegrees = newCompassTarget + (yawDegrees - offsetG);
// NSLog(@"Heading is available");
} else {
// NSLog(@"Heading isn't available");
}
if(yawDegrees < 0) {
yawDegrees = yawDegrees + 360;
}
newRotation = yawDegrees;
i++;
float gyroDegrees = (yawDegrees*radianConst);
if(updateCompass) {
[UIView beginAnimations:nil context:NULL];
[UIView setAnimationDuration:0.25];
[UIView setAnimationCurve:UIViewAnimationCurveEaseInOut];
[rotateImg setTransform:CGAffineTransformMakeRotation(-gyroDegrees)];
[UIView commitAnimations];
updateCompass = 0;
} else {
rotateImg.transform = CGAffineTransformMakeRotation(-gyroDegrees);
}
if(newRotation >350){
currentRotation=newRotation;
if (!updateTooLong) updateTooLong =
[NSTimer scheduledTimerWithTimeInterval:10 target:self
selector:@selector(tooLong:) userInfo:nil repeats:NO];
}
else if(newRotation <10){
currentRotation=newRotation;
if (!updateTooLong) updateTooLong =
[NSTimer scheduledTimerWithTimeInterval:10 target:self
selector:@selector(tooLong:) userInfo:nil repeats:NO];
}
else if(newRotation <30){
[self playSound];
}
else if(newRotation>120 ){
if (newRotation<240) {
[self halfWay];
}
}
}];
} else {
}
}
#pragma mark - Accelerometer
CGRect parentBounds = self.view.bounds;
const int tileW = parentBounds.size.width;
const int tileH = parentBounds.size.height;
if (!motionManager) motionManager = [[CMMotionManager alloc] init];
if (!currentQueue) currentQueue = [[NSOperationQueue alloc] init];
motionManager.deviceMotionUpdateInterval = 1.0/8.0;
if(motionManager.isDeviceMotionAvailable) {
[motionManager startDeviceMotionUpdatesToQueue:[NSOperationQueue currentQueue]
withHandler: ^(CMDeviceMotion *motion, NSError *error)
{
if(i > 6){
if(j > 0){
[self playSound];
countdownTimer = [NSTimer scheduledTimerWithTimeInterval: 0 target: self
selector: @selector(handleTimerTick) userInfo: nil repeats: NO];
}
currentJump = newJump;
j=0;
i=0;
}
CMAcceleration userAcceleration = motion.userAcceleration;
CMAcceleration gravity = motion.gravity;
float zValue = userAcceleration.y+gravity.y;
newJump= zValue;
i++;
self.horImg.center = CGPointMake(0+ (tileW/2), -zValue*100+ (tileH/2));
if(newJump>0.5){
j++;
}
else {
}
}];
} else {
}
}
#pragma mark - View lifecycle
- (void)viewDidLoad
{
[self doCountdown];
[super viewDidLoad];
NSURL *url = [NSURL fileURLWithPath:@"/dev/null"];
NSDictionary *settings = [NSDictionary dictionaryWithObjectsAndKeys:
[NSNumber numberWithFloat: 44100.0], AVSampleRateKey,
[NSNumber numberWithInt: kAudioFormatAppleLossless], AVFormatIDKey,
[NSNumber numberWithInt: 1], AVNumberOfChannelsKey,
[NSNumber numberWithInt: AVAudioQualityMax], AVEncoderAudioQualityKey,
nil];
NSError *error;
recorder = [[AVAudioRecorder alloc] initWithURL:url settings:settings error:&error];
if (recorder) {
[recorder prepareToRecord];
recorder.meteringEnabled = YES;
[recorder record];
levelTimer = [NSTimer scheduledTimerWithTimeInterval: 0.02 target: self
selector: @selector(levelTimerCallback:) userInfo: nil repeats: YES];
}
}
- (void)levelTimerCallback:(NSTimer *)timer {
if(i > 10){
if(j > 0){
countdownTimer = [NSTimer scheduledTimerWithTimeInterval: 0 target: self
selector: @selector(handleTimerTick) userInfo: nil repeats: NO];
}
j=0;
i=0;
}
CGRect parentBounds = self.view.bounds;
const int tileW = parentBounds.size.width;
[recorder updateMeters];
const double ALPHA = 0.05;
double peakPowerForChannel = pow(10, (0.05 * [recorder peakPowerForChannel:0]));
lowPassResults = ALPHA * peakPowerForChannel + (1.0 - ALPHA) * lowPassResults;
self.horImg.center = CGPointMake(0+ (tileW/2), -lowPassResults*400+ (440));
i++;
if (lowPassResults > 0.95){
j++;
}
}
#pragma mark - LocationManager
-(void)updateMotion;{
if (!locationManager) locationManager=[[CLLocationManager alloc] init];
locationManager.desiredAccuracy = kCLLocationAccuracyBest;
locationManager.delegate=self;
[locationManager startUpdatingHeading];
}
- (void)locationManager:(CLLocationManager *)manager didUpdateHeading:(CLHeading *)newHeading;
{
updatedHeading = newHeading.magneticHeading;
if(updatedHeading >350){
[self doCountdown];
[self toggleFlashlight];
[updateTooLong invalidate];
updateTooLong = nil;
}
else if(updatedHeading <10){
[self doCountdown];
[self toggleFlashlight];
[updateTooLong invalidate];
updateTooLong = nil;
}
else{
countLabel3.text = @"60 seconds";
[countdownTimer invalidate];
countdownTimer = nil;
if (!updateTooLong) updateTooLong = [NSTimer scheduledTimerWithTimeInterval:10 target:self
selector:@selector(tooLong:) userInfo:nil repeats:NO];
}
float headingFloat = 0 - newHeading.magneticHeading;
trueNorth.transform = CGAffineTransformMakeRotation(headingFloat*radianConst);
}
#pragma mark - Torch
-(void)handleTimerTick;
{
[self toggleFlashlight];
countdownTimer = [NSTimer scheduledTimerWithTimeInterval:1.0 target:self
selector:@selector(handleTimerTick) userInfo:nil repeats:NO];
}
- (void)toggleFlashlight;
{
AVCaptureDevice *device = [AVCaptureDevice defaultDeviceWithMediaType:AVMediaTypeVideo];
if([device hasTorch] == NO)
{
// NSLog(@"Error: This device doesnt have a torch");
}
if([device isTorchModeSupported:AVCaptureTorchModeOn] == NO)
{
// NSLog(@"Error: This device doesnt support AVCaptureTorchModeOn");
}
else{
[device lockForConfiguration:nil];
[device setTorchMode:AVCaptureTorchModeOn];
[device setTorchMode:AVCaptureTorchModeOff];
[device setTorchMode:AVCaptureTorchModeOn];
[device setTorchMode:AVCaptureTorchModeOff];
[device setTorchMode:AVCaptureTorchModeOn];
[device setTorchMode:AVCaptureTorchModeOff];
}
}
<Scheme
version = "1.3">
<BuildAction
parallelizeBuildables = "YES"
buildImplicitDependencies = "YES">
<BuildActionEntries>
<BuildActionEntry
buildForTesting = "YES"
buildForRunning = "YES"
buildForProfiling = "YES"
buildForArchiving = "YES"
buildForAnalyzing = "YES">
<BuildableReference
BuildableIdentifier = "primary"
BlueprintIdentifier = "EBEBA7071494B17F001F76C3"
BuildableName = "ZYX.app"
BlueprintName = "ZYX"
ReferencedContainer = "container:ZYX.xcodeproj">
</BuildableReference>
</BuildActionEntry>
</BuildActionEntries>
</BuildAction>
<LaunchAction
selectedDebuggerIdentifier = "Xcode.DebuggerFoundation.Debugger.GDB"
selectedLauncherIdentifier = "Xcode.DebuggerFoundation.Launcher.GDB"
launchStyle = "0"
useCustomWorkingDirectory = "NO"
buildConfiguration = "Release"
debugDocumentVersioning = "YES"
allowLocationSimulation = "YES">
<BuildableProductRunnable>
<BuildableReference
BuildableIdentifier = "primary"
BlueprintIdentifier = "EBEBA7071494B17F001F76C3"
BuildableName = "ZYX.app"
BlueprintName = "ZYX"
ReferencedContainer = "container:ZYX.xcodeproj">
</BuildableReference>
</BuildableProductRunnable>
<AdditionalOptions>
</AdditionalOptions>
</LaunchAction>
<ProfileAction
shouldUseLaunchSchemeArgsEnv = "YES"
savedToolIdentifier = ""
useCustomWorkingDirectory = "NO"
buildConfiguration = "Release"
debugDocumentVersioning = "YES">
<BuildableProductRunnable>
<BuildableReference
BuildableIdentifier = "primary"
BlueprintIdentifier = "EBEBA7071494B17F001F76C3"
BuildableName = "ZYX.app"
BlueprintName = "ZYX"
ReferencedContainer = "container:ZYX.xcodeproj">
</BuildableReference>
</BuildableProductRunnable>
</ProfileAction>
<AnalyzeAction
buildConfiguration = "Debug">
</AnalyzeAction>
<ArchiveAction
buildConfiguration = "Release"
customArchiveName = "JODI"
revealArchiveInOrganizer = "YES">
</ArchiveAction>
</Scheme>
Use the Resolution Center to correspond with App Review until all issues with your app version have been resolved. Binary Rejected Mar 1, 2012 03:11 PM Reasons for Rejection: • 10.6: Apple and our customers place a high value on simple, refined, creative, well thought through interfaces. They take more work but are worth it. Apple sets a high bar. If your user interface is complex or less than very good it may be rejected Mar 1, 2012 03:11 PM. From Apple. 10.6 We found the user interface of your app is not of sufficient quality to be appropriate for the App Store. Apps that provide a poor user experience are not in compliance with the App Store Review Guidelines. Specifically, we noticed the user interface of your app is not intuitive and could not be navigated. For example, it asks the user to turn right ten times. Simply turning the phone to the right did not increase the count. After moving the phone in several directions it began counting, but repeating the same task would only allow the count to go up to 4, at which point it looped back to provide the same command to 'turn right ten times'. Please evaluate whether you can make the necessary revisions to improve the user experience of your app.
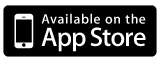
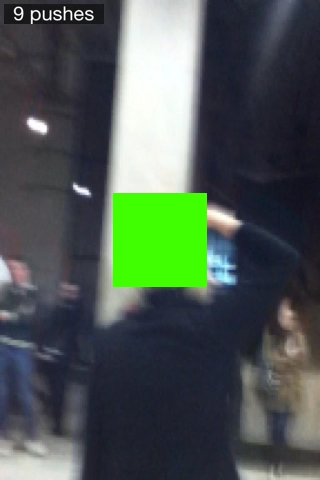
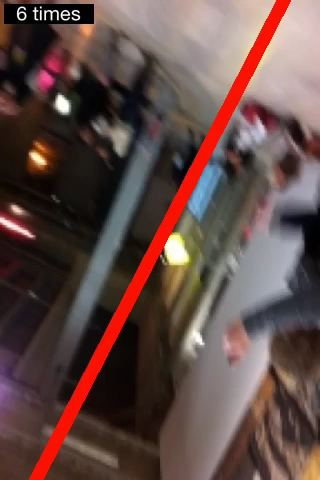
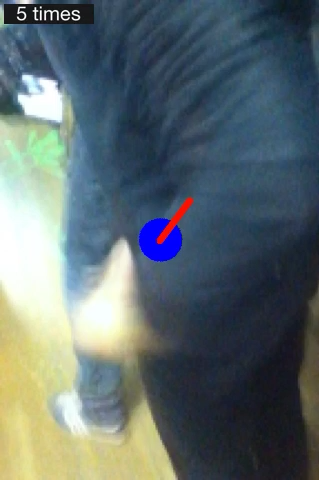
"ZYX uses the iPhone or iPod Touch's built-in motion-tracking capabilities to guide users through a series of gestures, from turning in a circle to raising one's arm up and down. Each time a gesture is performed correctly, the phone clicks; when
all gestures have been completed, the device sounds an alarm in celebration. This app situates the user in a realm that is both virtual and physical. Bystanders see the user as performing a strange dance; in contrast, the iPhone observes and rewards the user's adherence to a prescribed set of movements. This dissonance between the virtual space inhabited by the iPhone user and the physical space occupied by the observer has become an everyday phenomenon, exemplified by the experience of passing someone on the street who appears to be delivering a nonsensical monologue while speaking into the microphone of a wireless mobile device."